Node Redis: Delete Keys By Prefix (Detailed Guide w/ Code Examples)
Use Case(s)
Often times, Redis is used as a cache and it becomes necessary to delete keys based on certain patterns or prefixes. For example, you might want to invalidate all cache entries that have a specific prefix when an update occurs.
Code Examples
The 'redis' module in Node.js doesn't directly support deleting keys by prefix. But you can achieve this by combining the 'KEYS' command and the 'DEL' command.
const redis = require('redis');
const client = redis.createClient();
client.keys('prefix:*', (err, keys) => {
if (err) throw err;
client.del(keys, (err, reply) => {
console.log(reply);
});
});
In this code, we first obtain all keys with the provided prefix using the 'keys' function and then delete them using the 'del' function.
Best Practices
- Be careful when using the KEYS command in a production environment because it can significantly impact performance when there are lots of keys.
- It's better to use SCAN instead, which is a cursor-based iterator and doesn't block the database.
Common Mistakes
- Not handling errors properly can lead to unexpected behavior.
- Using the KEYS command in a production environment can degrade performance.
FAQs
Q: Can I use wildcard characters in the key prefix? A: Yes, you can use wildcard characters like '*' in the prefix to match multiple keys.
Q: What happens when no keys match the prefix? A: The del
command will not delete any keys, and it will return 0.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
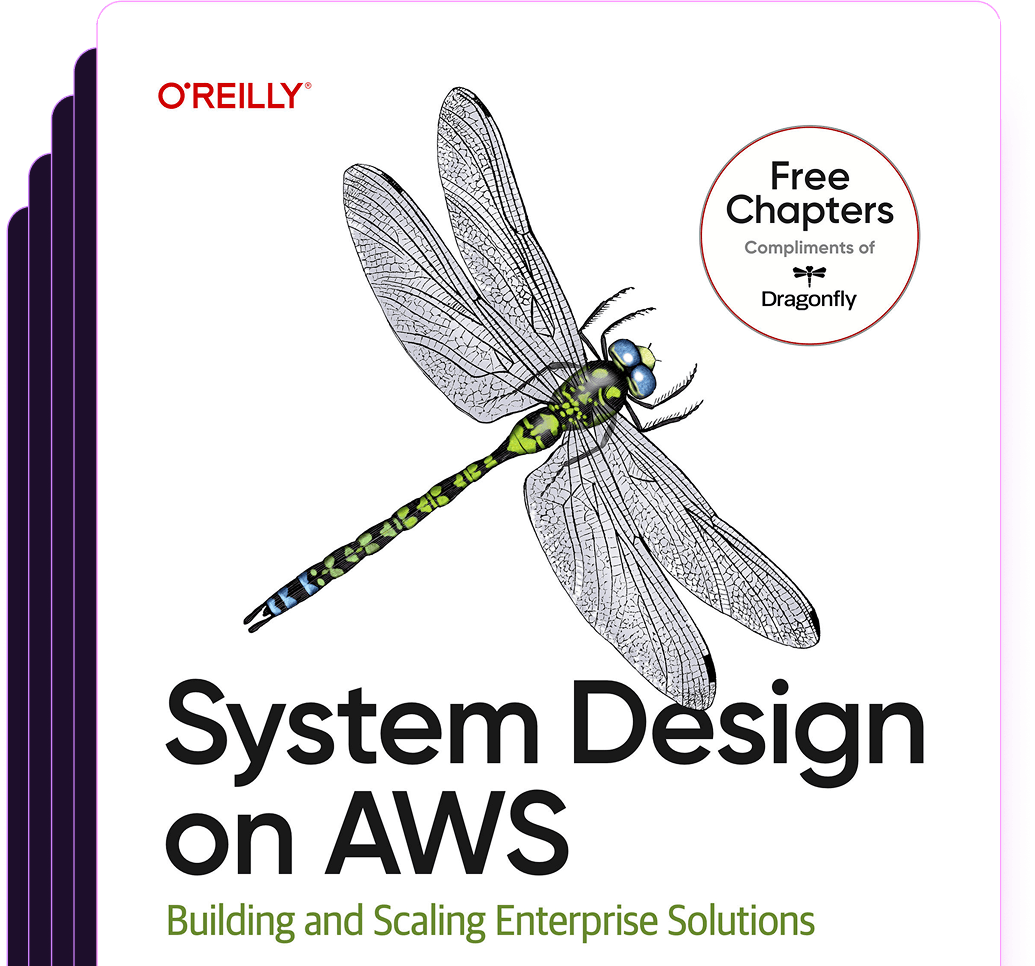
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost