Getting a List in Node.js using Redis (Detailed Guide w/ Code Examples)
Use Case(s)
Retrieving a list from Redis is a common use case when you need to get an array of elements saved previously. These lists can serve as message queues, timelines, or even just a simple stack or queue.
Code Examples
The following example demonstrates how to retrieve a list in Node.js using the redis
module:
```
const redis = require('redis');
const client = redis.createClient();
client.lrange('mylist', 0, -1, function(err, reply) {
console.log(reply);
});
```
In this code:
- 'mylist' is the key associated with the list in Redis.
- 0 and -1 specify the range of elements to retrieve from the list. In this case, it retrieves all elements in the list.
Another example shows how to get only the first three items from the list:
```
client.lrange('mylist', 0, 2, function(err, reply) {
console.log(reply);
});
```
Best Practices
- Make sure that the key you're trying to retrieve actually corresponds to a list. If it's not, you'll get an error.
- You should handle any possible errors in the callback to prevent crashes in your application.
Common Mistakes
- Not understanding that Redis lists are 0-indexed and inclusive. When you do
lrange('mylist', 0, 2)
, it includes the element at index 2.
FAQs
Q: What does -1 mean in the lrange command?
A: In the context of the Redis lrange
command, -1 means 'until the end of the list'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
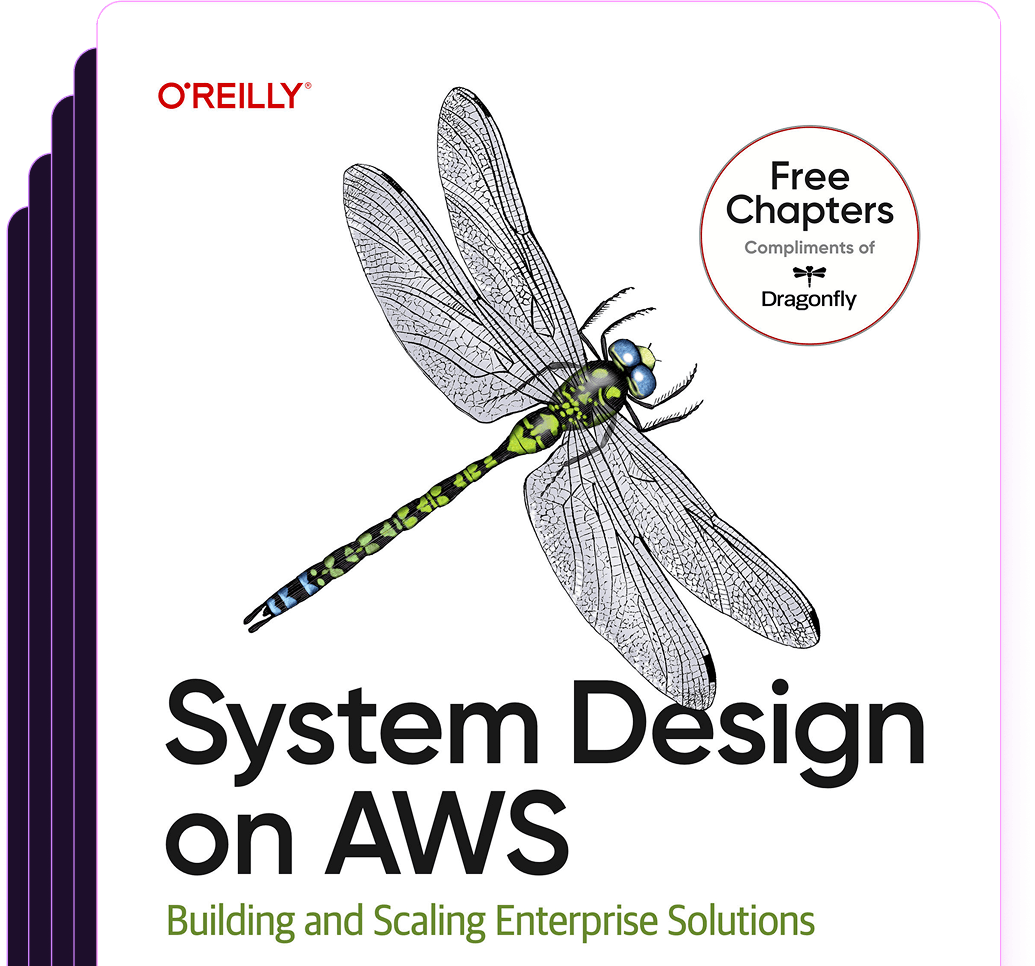
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost