Node Redis: Get Multiple Keys (Detailed Guide w/ Code Examples)
Use Case(s)
MGET
is a command in Redis that allows you to retrieve multiple keys in a single operation, which can be useful when you need to fetch many values simultaneously, improving the efficiency over separate GET
operations.
Code Examples
Example 1: Basic usage of MGET
with Node.js and redis package.
const redis = require('redis');
const client = redis.createClient();
client.mget(['key1', 'key2', 'key3'], function(err, reply) {
console.log(reply); // Prints an array of values for 'key1', 'key2', and 'key3'
});
In this example, we create a Redis client and use the mget
method to get the values of 'key1', 'key2', and 'key3'. The result is an array containing the value of each key.
Example 2: Handling missing keys.
const redis = require('redis');
const client = redis.createClient();
client.mget(['missingKey', 'anotherMissingKey'], function(err, reply) {
console.log(reply); // Prints: [ null, null ]
});
In this example, if a key doesn't exist in the Redis store, mget
returns null
for that key.
Best Practices
- Use
MGET
over individualGET
commands when you need to retrieve multiple keys at once. It reduces network latency and improves performance.
Common Mistakes
- Not checking for
null
values in the result array.MGET
returnsnull
for keys that do not exist.
FAQs
Q: Can I mix existing and non-existing keys while using MGET?
A: Yes, MGET
will return an array with the values of existing keys and null
for non-existing keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
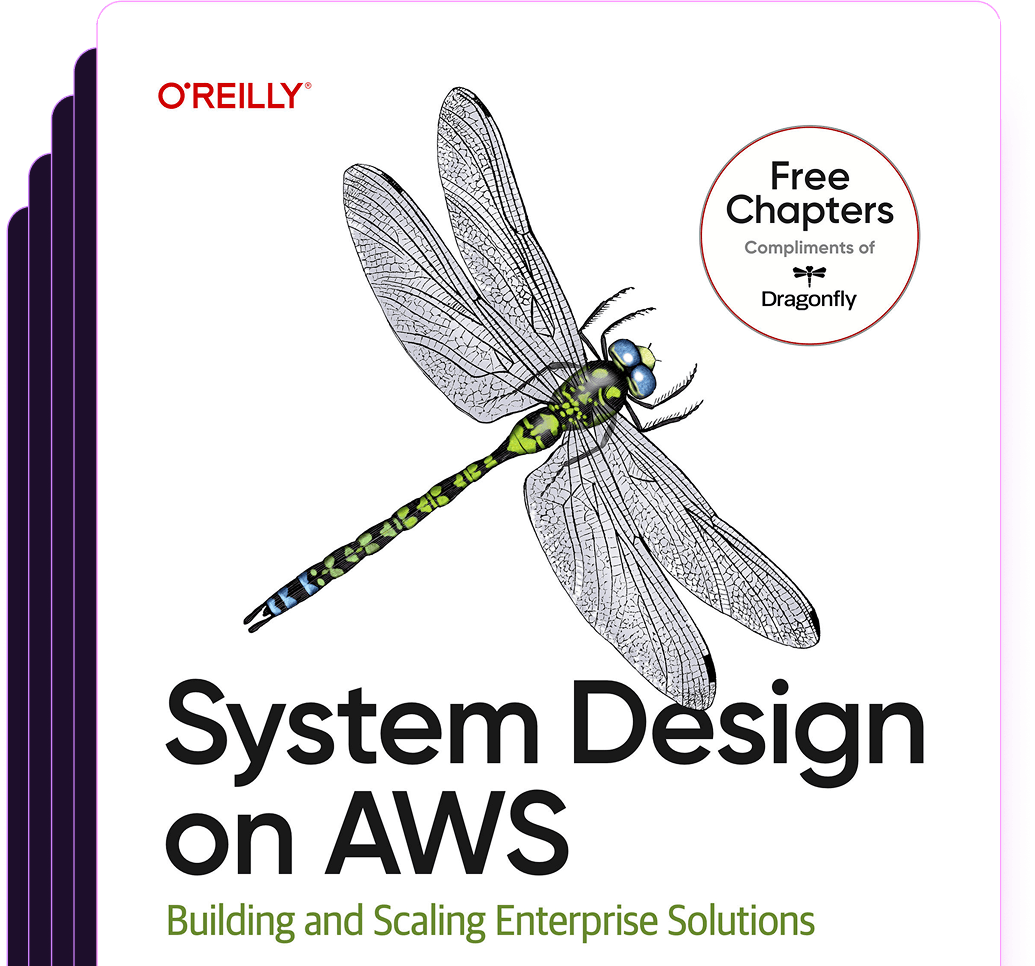
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost