Getting all Redis keys starting with a specific pattern in Python (Detailed Guide w/ Code Examples)
Use Case(s)
It's common to use key naming schemes in Redis databases where related keys share a common prefix. This allows us to retrieve all the keys related to a particular subset or type of data. It's useful when you need to perform batch operations on these keys.
Code Examples
The keys
function in Redis library for Python can be used to fetch all keys that match a certain pattern.
Example:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
keys = r.keys('prefix*')
for key in keys:
print(key.decode('utf-8'))
In this example, we connect to a local Redis server and get all keys that start with 'prefix'. The keys are returned as bytes, so we decode them to UTF-8 strings before printing.
Best Practices
- While you can certainly use the
keys
function to fetch all keys matching a pattern, it's not recommended for production environments especially when dealing with large datasets. This is because thekeys
command can block the Redis server while it's executing, which could lead to performance issues. - For production purposes, you should use the
scan
function, which is non-blocking and can iterate over the keyspace without affecting the performance of the server.
Common Mistakes
A common mistake is to forget decoding the keys after getting them from Redis. They are returned as bytes, so remember to decode them to UTF-8 strings before use.
FAQs
Q: What are the alternatives to using the keys function in Redis? A: You can use the scan
function, which provides a cursor based iterator that doesn't block the server like the keys command.
Q: Why does the Redis keys command return keys in bytes? A: Redis is designed to be language agnostic and it returns data in bytes because it's a common format that can be interpreted by any language.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
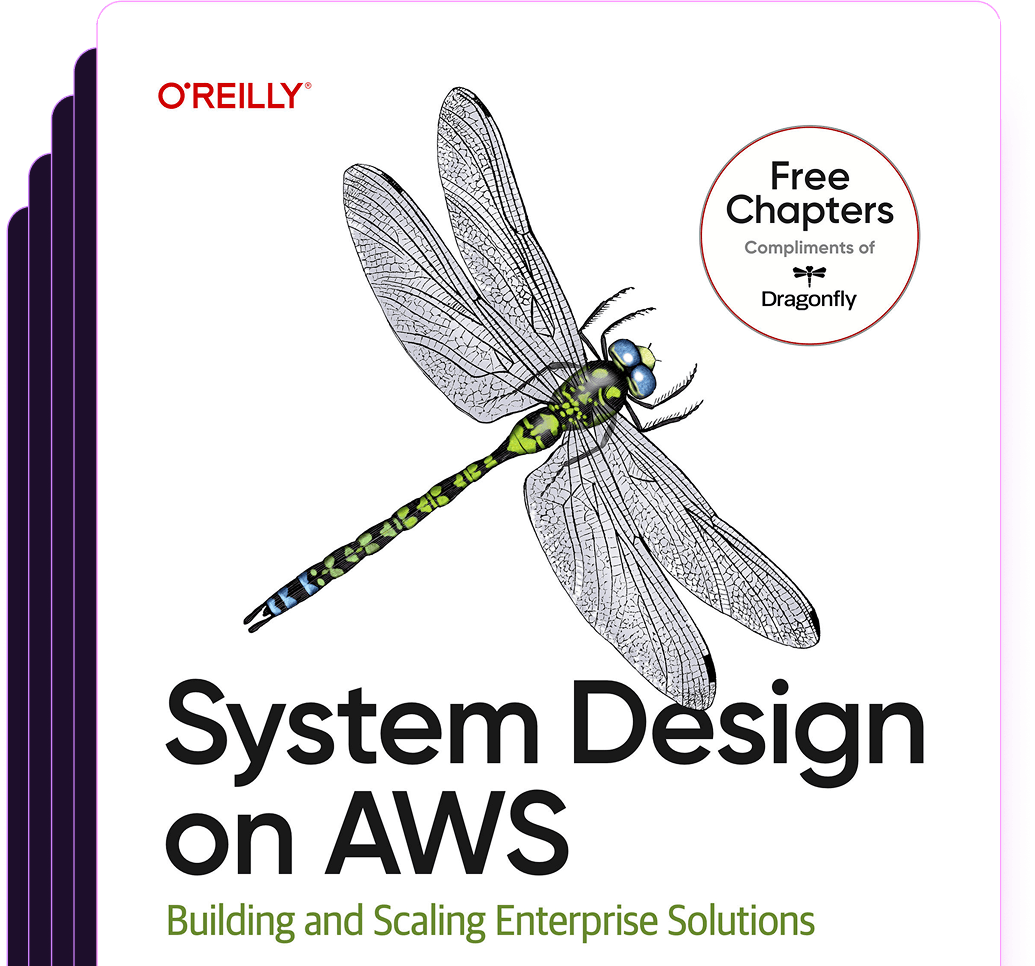
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost