Getting Key Type in Redis using Python (Detailed Guide w/ Code Examples)
Use Case(s)
The 'TYPE' command in Redis is commonly used when you need to know the type of the key-value pair stored inside your Redis database. This can be helpful when debugging, or when dealing with a database where the data types aren't well-documented.
Code Examples
Let's assume you have installed redis-py
library and established a connection to your Redis server. Here’s how you can get the type of a specific key:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
key_type = r.type('your_key')
print(key_type) # Outputs: set, list, zset, hash, string, or none (if key does not exist)
In this example, we're connecting to a Redis server running on localhost and then we use the type()
method provided by redis-py
to get the type of the key named 'your_key'. The returned value will be the data type of the key ('set', 'list', 'zset', 'hash', 'string') or 'none' if the key does not exist.
Best Practices
- Always check if a key exists before trying to get its type to avoid unnecessary operations.
- Make sure to handle the case when the key does not exist or its type is 'none'.
Common Mistakes
- Not handling the case when the key does not exist, which might lead to incorrect assumptions about the data.
- Misunderstanding the output: The command does not return Python types, but Redis types.
FAQs
- What does 'none' mean when using the type command in Redis? - 'None' indicates that the key does not exist.
- Does the type command work with all Redis data structures? - Yes, it provides the type for all standard Redis data structures (string, list, set, sorted set, and hash).
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
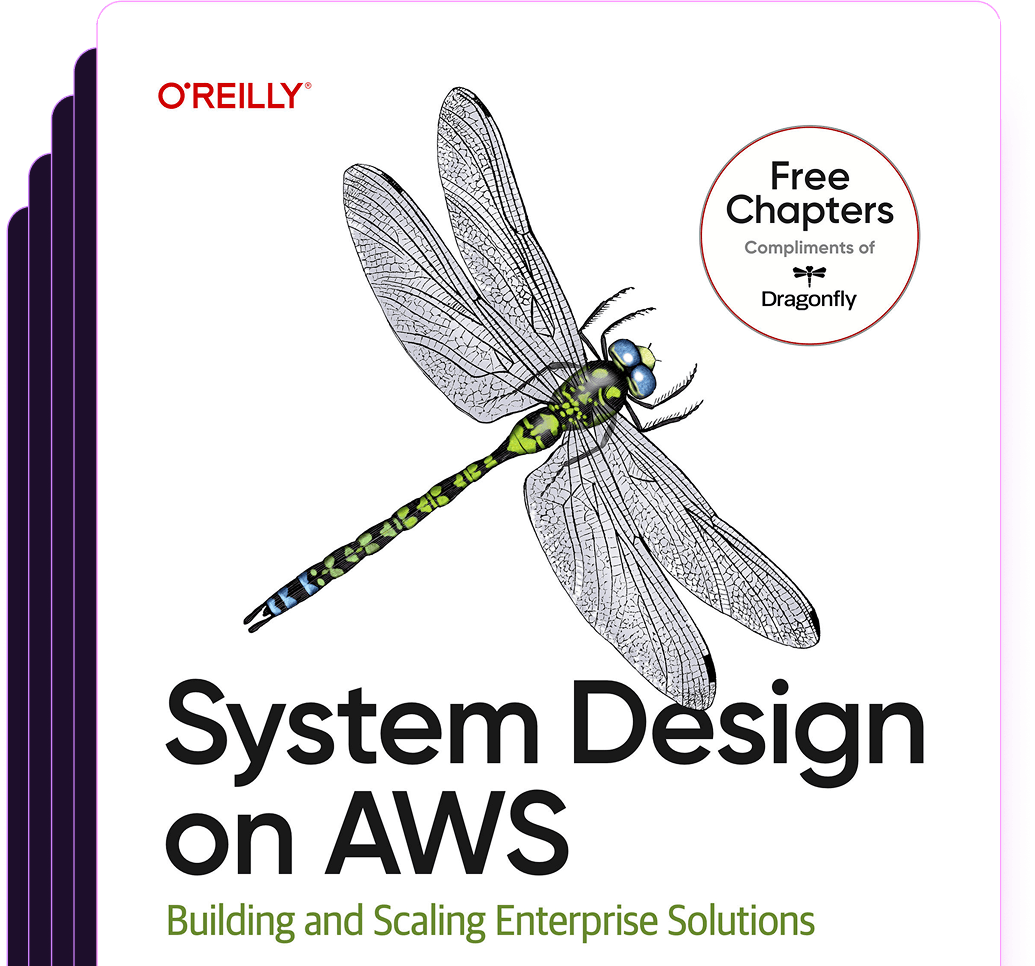
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost