Getting Last Update Time in Redis using Python (Detailed Guide w/ Code Examples)
Use Case(s)
The last update time in Redis is often required when it comes to synchronization or cache invalidation. This information can help in understanding when a key-value pair was last modified and hence, whether the data is stale or not.
Code Examples
Please note that Redis itself does not provide direct commands to fetch the last update time of a key. However, we can maintain this information ourselves within our Python code. Here is how you might do this:
Example 1: Manual tracking of last update time
import redis
from datetime import datetime
r = redis.Redis(host='localhost', port=6379, db=0)
def set_key(key, value):
r.set(key, value)
r.set(f'{key}_last_updated', datetime.now().isoformat())
def get_last_update(key):
return r.get(f'{key}_last_updated')
In this example, every time a key is set, another key with the same name appended by _last_updated
is also set with the current timestamp. To get the last updated time, simply fetch this key.
Best Practices
- Using an appropriate naming scheme for your keys will make it easier to correlate keys with their last updated timestamp keys.
- Ensure proper error handling around the Redis calls as network issues or Redis server issues could lead to exceptions.
Common Mistakes
- A common mistake is forgetting to set the 'last update' time whenever updating a key. Make sure that the timestamp is updated every time the corresponding data is changed.
FAQs
- Does Redis provide a built-in feature for this? No, Redis does not have a built-in feature to get the last update time of a key. It needs to be manually tracked.
- What happens when setting a non-existent timestamp key? If you try to retrieve a non-existent timestamp key, Redis will return None.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Redis: Get Key Where Value in Python
- Python: Redis Get Keys Without TTL
- Getting Redis Replicas in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
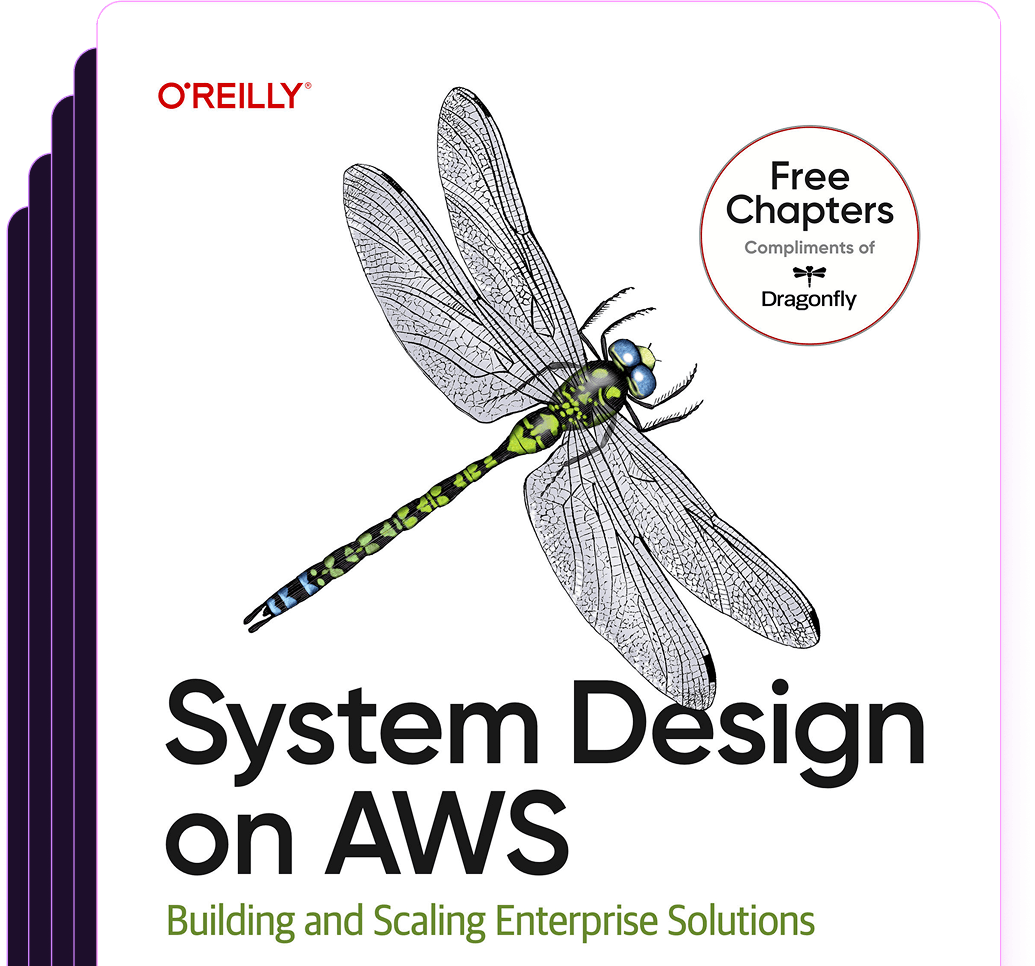
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost