Retrieving Multiple Values from Redis in Python (Detailed Guide w/ Code Examples)
Use Case(s)
In Python, retrieving multiple values from Redis is a common operation when you have to process or analyze a group of related data elements at once. This can be particularly useful in applications that need to fetch user profiles, configuration settings, session information, or other groups of related data.
Code Examples
Example 1: Retrieve multiple keys using mget()
Here's how to use the mget()
function in Redis-py:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
keys = ['key1', 'key2', 'key3']
values = r.mget(keys)
print(values)
In this code, we first establish a connection with the Redis server. Then we define a list of keys for which we want to retrieve the values. The mget()
function retrieves the values associated with each key in the list.
Example 2: Handle non-existent keys with mget()
It's important to note that mget()
will return None for any key that does not exist:
non_existent_keys = ['key4', 'key5']
values = r.mget(non_existent_keys)
print(values) # Outputs: [None, None]
Here, 'key4' and 'key5' do not exist in the Redis database, so mget()
returns None for these keys.
Best Practices
- Always check for
None
in the result list after callingmget()
, as it signifies that the corresponding key did not exist in the Redis database. - Use
mget()
instead of multipleget()
calls to reduce network latency and improve application performance.
Common Mistakes
- Not handling None values returned by
mget()
can lead to unexpected behavior or errors.
FAQs
Q: What does mget()
return if a key doesn't exist in the database?
A: mget()
will return None for any key that does not exist in the Redis database.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
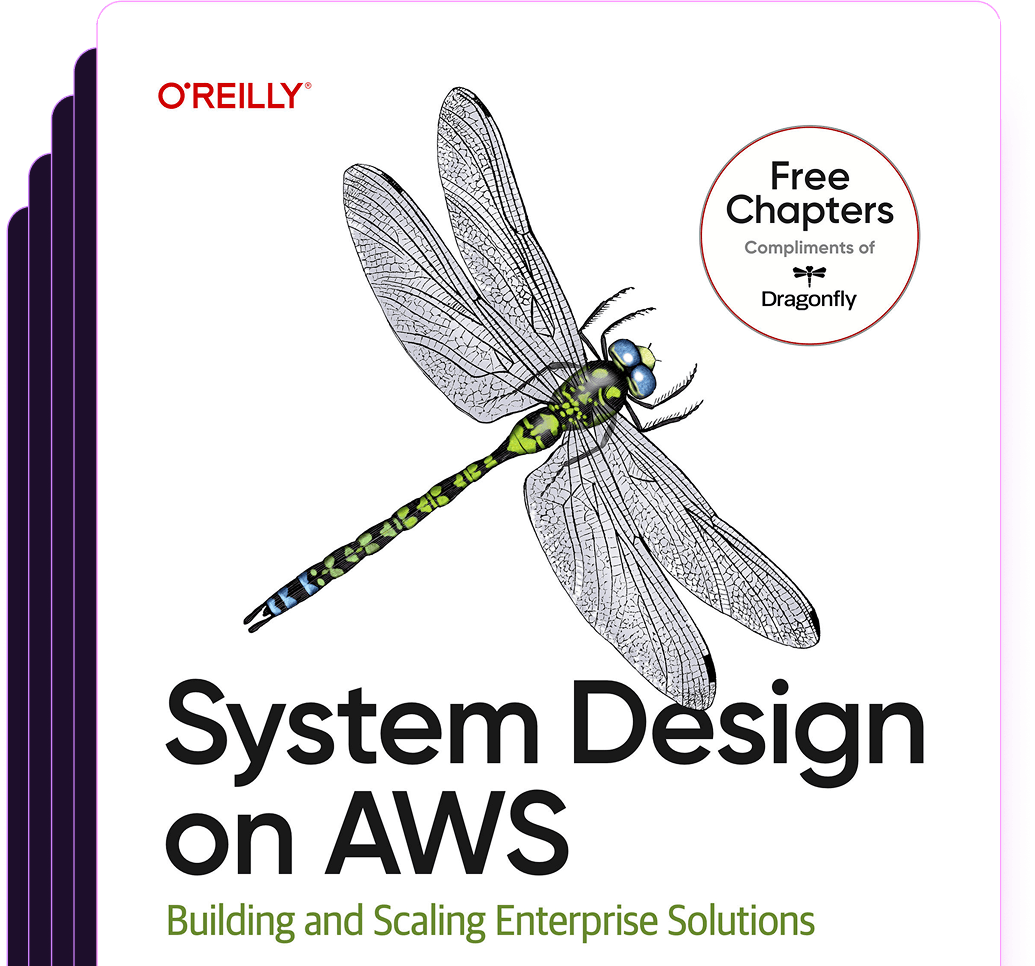
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost