Working with Redis Lists in Python (Detailed Guide w/ Code Examples)
Use Case(s)
Redis lists can be used as a simple queue for tasks or to store a list of items, such as user activities on a website. In Python, getting elements from a Redis list is a common operation.
Code Examples
Example 1: Getting all elements from a Redis List
Using the LRANGE
command, you can retrieve all elements from a Redis List. Here's how you do it in Python using redis-py:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
all_items = r.lrange('mylist', 0, -1)
print(all_items)
In this example, 'mylist' is the name of your list, and 0 and -1 are the start and stop parameters which indicate that we want to get all items from the list.
Example 2: Pop an item from a Redis List
You might want to pop an item from a list (essential for using a list as a queue). You can do this using LPOP
or RPOP
:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
popped_item = r.lpop('mylist')
print(popped_item)
Here, lpop
pops and returns the first item from the list named 'mylist'.
Best Practices
- Use the
BLPOP
orBRPOP
commands if you want to pop an element from a list but the list could be empty. These commands block the connection until another client pushes an item to the list. - Be aware of the big-O notation for list operations in Redis. For instance, 'LRANGE' is O(S+N) where S is the distance of start offset from HEAD for small lists, from nearest end (HEAD or TAIL) for large lists; and N is the number of elements in the specified range.
Common Mistakes
- A common mistake when using
LRANGE
is misunderstanding the start and stop parameters. They are zero-based indexes, with 0 being the first element of the list, 1 being the second element, and so on. Negative indices count from the end of the list.
FAQs
Q: What happens if I try to pop an item from an empty list using redis-py?
A: If you use LPOP
or RPOP
, it will return None. However, if you use BLPOP
or BRPOP
, it will block the client until an item is pushed.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Getting All Keys Matching a Pattern in Redis using Python
- Getting the Length of a Redis List in Python
- Getting Server Info from Redis in Python
- Getting Number of Connections in Redis Using Python
- Getting Current Redis Version in Python
- Getting Memory Stats in Redis using Python
- Redis Get All Databases in Python
- Redis Get All Keys and Values in Python
- Retrieving a Key by Value in Redis Using Python
- Python Redis: Get Config Settings
- Getting All Hash Keys Using Redis in Python
- Get Total Commands Processed in Redis Using Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
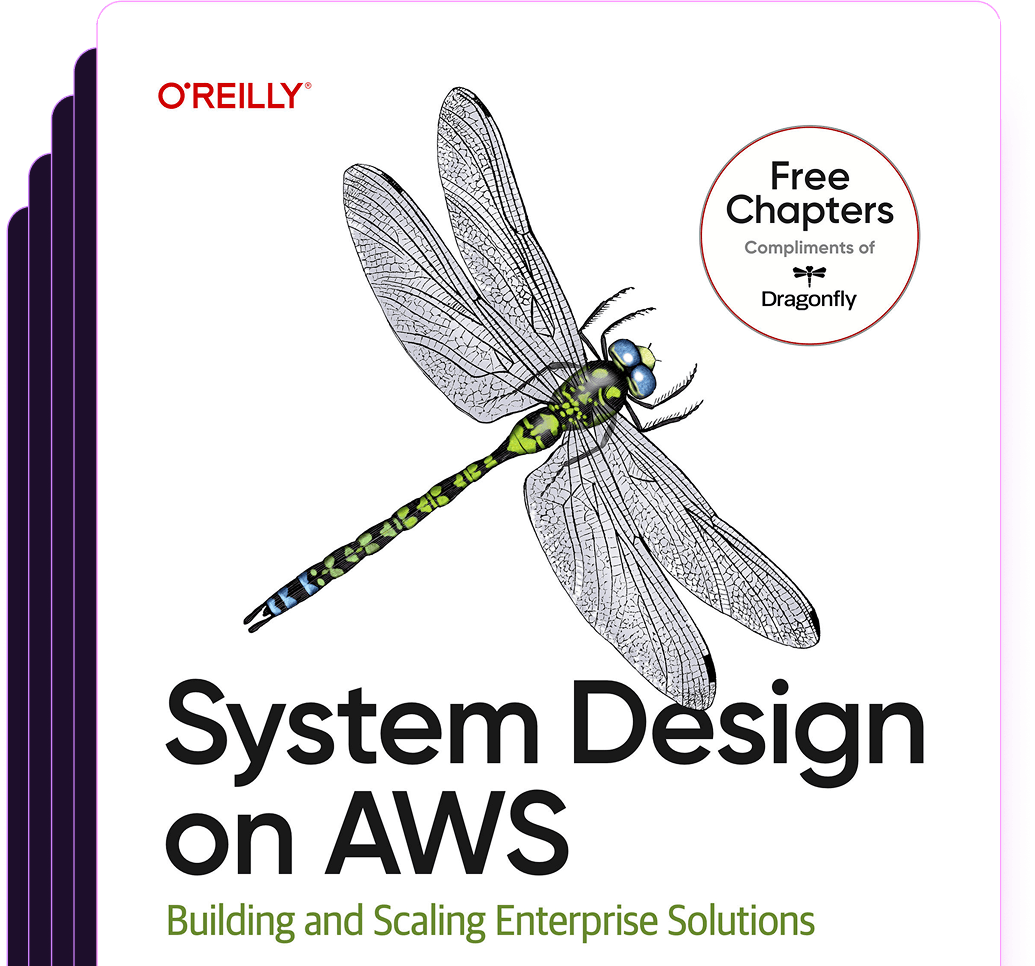
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost