Question: How to Connect to ElastiCache Redis
Answer
To connect to an ElastiCache Redis cluster, you need to do the following steps:
- Find the Cluster Endpoint: Locate the endpoint address of the ElastiCache Redis cluster in the Amazon Web Services (AWS) Management Console.
- Configure your Local Environment: Ensure that you have the necessary tools and dependencies installed on your local machine or application server.
- Establish a Connection: Use a Redis client to connect to the ElastiCache Redis cluster using the endpoint address.
Here's a step-by-step guide with code examples:
1. Find the Cluster Endpoint
Navigate to the ElastiCache section of the AWS Management Console to locate your Redis cluster. Click on the cluster name, and find the "Primary Endpoint" under the details tab.
2. Configure your Local Environment
Ensure you have redis-cli
installed on your local machine or application server. For example, on Ubuntu, you can use the following command to install it:
sudo apt-get install redis-tools
For Python applications, you will need to install the redis
package:
pip install redis
3. Establish a Connection
Using redis-cli
:
Connect to the ElastiCache Redis cluster with the redis-cli
tool:
redis-cli -h <REDIS_HOST> -p <REDIS_PORT>
Replace <REDIS_HOST>
and <REDIS_PORT>
with your cluster's endpoint address and port, respectively.
Using Python:
In a Python script, use the redis
library to connect to the ElastiCache Redis cluster:
import redis
# Replace <REDIS_HOST> and <REDIS_PORT> with your cluster's endpoint address and port, respectively.
redis_host = "<REDIS_HOST>"
redis_port = <REDIS_PORT>
r = redis.Redis(host=redis_host, port=redis_port)
# Test the connection
response = r.ping()
print("Connected to Redis:", response)
Now that you've successfully connected to your ElastiCache Redis cluster, you can perform operations like reading and writing data using the Redis commands available in your chosen client.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common ElastiCache Questions (and Answers)
- How to configure ElastiCache in AWS?
- How to view ElastiCache data?
- Is ElastiCache stateless?
- What is ElastiCache Replication Group?
- When to use ElastiCache?
- Can ElastiCache store session data?
- How to improve ElastiCache performance?
- Can't connect to ElastiCache Redis
- Is ElastiCache a database?
- How to clear Elasticache?
- How many ElastiCache nodes do I need?
- Does ElastiCache work with RDS?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
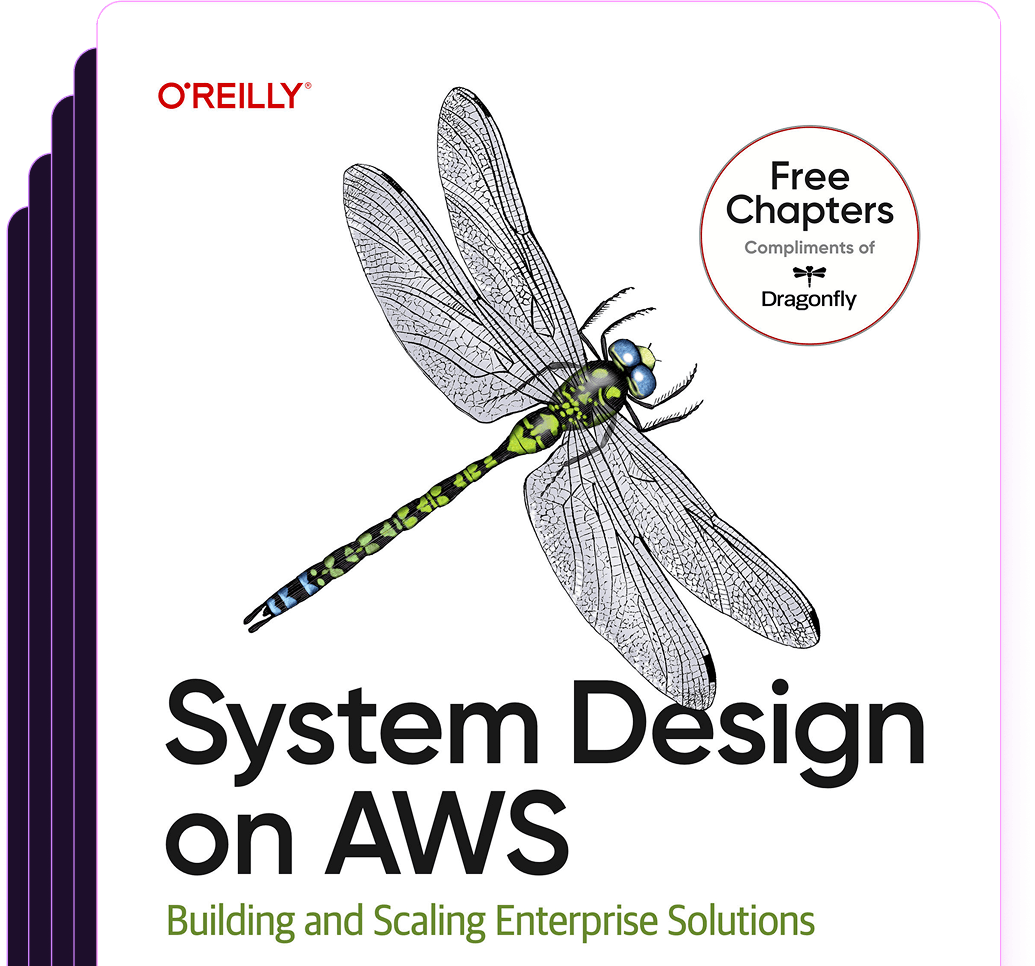
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost