Question: How to Connect to Redis ElastiCache from Node.js
Answer
To connect to a Redis ElastiCache cluster from a Node.js application, follow these steps:
- Install the
redis
package using npm:
```
npm install redis
```
- Import the
redis
package in your Node.js file and create a Redis client instance with the appropriate configuration options:
```javascript
const redis = require('redis');
// Replace the following values with your ElastiCache configuration details
const host = 'your-elasticache-endpoint-url';
const port = 6379; // Default Redis port
// Create the Redis client
const client = redis.createClient({ host, port });
```
- Configure error and connection event handlers for the Redis client:
```javascript
// Handle errors
client.on('error', (error) => {
console.error(Redis error: ${error}
);
});
// Connection event
client.on('connect', () => {
console.info('Connected to ElastiCache Redis');
});
```
- Use the Redis client to interact with your ElastiCache instance using available Redis commands:
```javascript
// Set a key-value pair in the cache
client.set('my_key', 'my_value', (err, response) => {
if (err) {
console.error(Set error: ${err}
);
} else {
console.log(Set response: ${response}
);
}
});
// Retrieve the value of the key from the cache
client.get('my_key', (err, value) => {
if (err) {
console.error(Get error: ${err}
);
} else {
console.log(Get value: ${value}
);
}
});
```
- Remember to close the connection when you're done using it:
```javascript
client.quit();
```
Here's a full example:
const redis = require('redis');
const host = 'your-elasticache-endpoint-url';
const port = 6379;
const client = redis.createClient({ host, port });
client.on('error', (error) => {
console.error(`Redis error: ${error}`);
});
client.on('connect', () => {
console.info('Connected to ElastiCache Redis');
});
client.set('my_key', 'my_value', (err, response) => {
if (err) {
console.error(`Set error: ${err}`);
} else {
console.log(`Set response: ${response}`);
}
});
client.get('my_key', (err, value) => {
if (err) {
console.error(`Get error: ${err}`);
} else {
console.log(`Get value: ${value}`);
}
});
// Close the connection when you're done
client.quit();
Make sure to replace your-elasticache-endpoint-url
with your actual ElastiCache cluster endpoint URL and customize the code as needed.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common ElastiCache Questions (and Answers)
- How to configure ElastiCache in AWS?
- How to view ElastiCache data?
- Is ElastiCache stateless?
- What is ElastiCache Replication Group?
- When to use ElastiCache?
- Can ElastiCache store session data?
- How to improve ElastiCache performance?
- Can't connect to ElastiCache Redis
- Is ElastiCache a database?
- How to clear Elasticache?
- How many ElastiCache nodes do I need?
- Does ElastiCache work with RDS?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
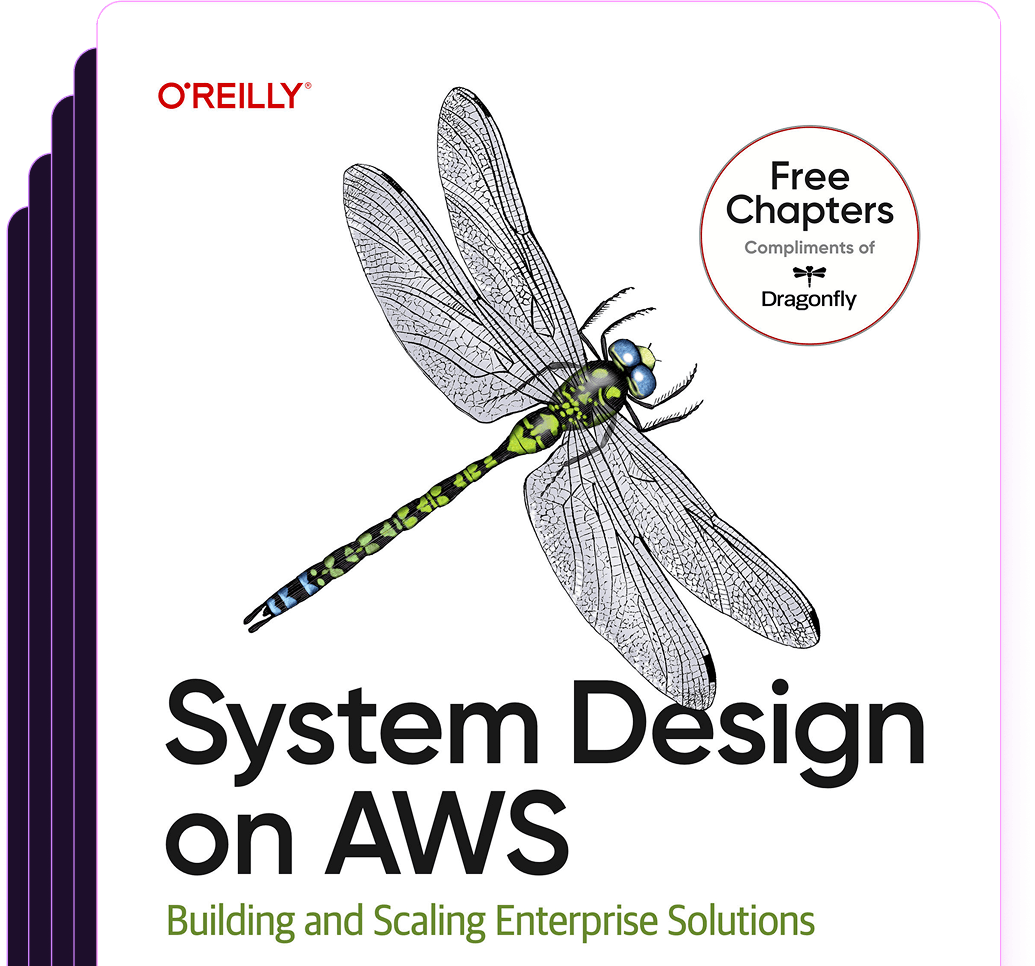
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost