Question: How can we implement a distributed LRU (Least Recently Used) cache?
Answer
Implementing a Distributed Least Recently Used (LRU) Cache involves managing data across multiple nodes to ensure scalability and high availability. It is based on the LRU caching scheme, which evicts the least recently used items first.
Here's a basic outline-solid of how you might do it:
1. Select an appropriate distributed cache system
The first step is to choose a distributed cache system that supports LRU eviction policy, like Redis or Memcached.
For example, let's take Redis. You need to enable the maxmemory-policy
to allkeys-lru
in your redis config file (redis.conf
). Here's how you can achieve this:
maxmemory 2gb # setting max memory usage to 2GB
maxmemory-policy allkeys-lru # setting eviction policy to LRU
2. Implement cache sharding
Cache sharding is essential for maintaining uniform load distribution across multiple cache instances. The most common way to implement sharding is using consistent hashing, which minimizes reorganization when nodes are added or removed.
To hash your keys, you can use SHA1 or MD5 hash functions, then map these hashed keys to your Redis nodes.
import hashlib
def get_server_node(key):
node_number = int(hashlib.md5(key.encode('utf-8')).hexdigest(), 16) % NUM_NODES
return "redis_node_" + str(node_number)
3. Set up data replication
To prevent data loss and improve read performance, set up data replication across the nodes. Redis provides support for primary-replica replication.
In your redis config file (redis.conf
), you can specify a master node like this:
replicaof <masterip> <masterport>
4. Implement client-side logic
Finally, you need to implement logic in your application to interact with your distributed cache. This includes connecting to the right node, handling failed nodes, and executing cache operations (get, set, delete, etc.).
For Python, you can use the redis-py package:
import redis
def get_from_cache(key):
# Determine the appropriate node
node = get_server_node(key)
# Connect to the node
r = redis.Redis(host=node, port=6379, db=0)
# Get the value from the cache
value = r.get(key)
return value
This is a very basic overview. There are many factors to consider when designing a distributed caching system, like error handling, network partitions, security, and more. Be sure to research thoroughly and test extensively.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- How can you implement a distributed cache using Docker?
- What are the differences between a centralized cache and a distributed cache?
- What is the best distributed cache for Java?
- What is the difference between distributed cache and local cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
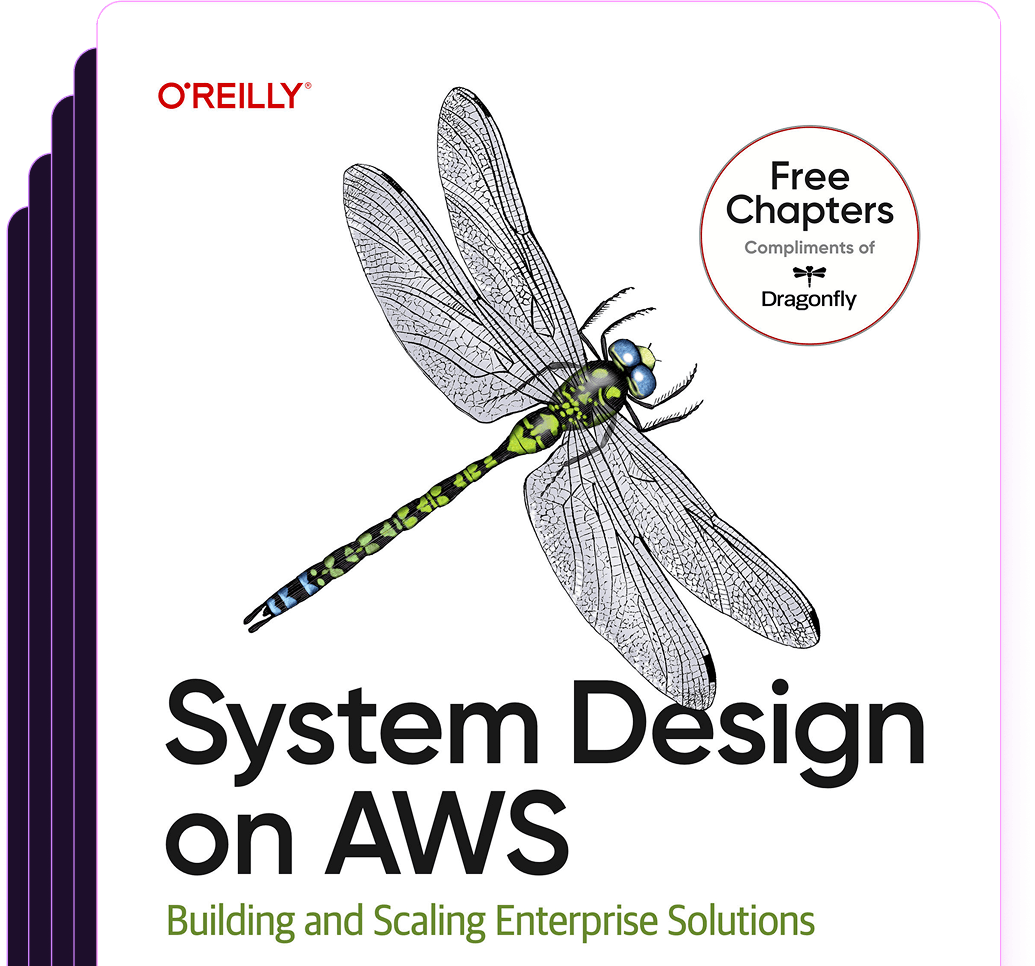
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost