Question: How can I implement in-memory caching in AWS Lambda?
Answer
AWS Lambda is a serverless computing service that runs your code in response to events and automatically manages the underlying compute resources for you. It allows developers to build scalable and efficient applications without having to manage servers.
In-memory caching can be used to improve the performance of AWS Lambda applications by storing data in memory between invocations, reducing the need for expensive database or API calls.
Here is an example of how you might use global variables in Node.js to store data in memory:
let myCache = {};
exports.handler = async (event) => {
const id = event.id;
if (myCache[id]) {
return myCache[id];
} else {
// assuming getData() is a function that fetches data from an API or database
const data = await getData(id);
myCache[id] = data;
return data;
}
};
This simple cache stores data in a JavaScript object named myCache
. When the Lambda function receives an event, it first checks if the needed data is already in the cache. If so, it immediately returns the cached data; otherwise, it fetches the data from another source (like a database or an API), stores it in the cache, and then returns it.
However, you should note that AWS Lambda may decide to freeze and later thaw your function for its internal resource management purposes. When a function is frozen, all processing stops until the function is thawed. Thawed functions can continue where they left off, which includes any in-memory data such as cached values.
Also, there's no guarantee about persistency of the cache between different lambda invocations, because AWS might choose to kill and recreate the container where your Lambda function runs. So it's reliable only inside single session.
For these reasons, while in-memory caching with AWS Lambda can increase performance, it should not be used as a replacement for persistent storage or used to store crucial data. Instead, consider using a dedicated caching service like Amazon ElastiCache or AWS DynamoDB's DAX (DynamoDB Accelerator), depending on your specific needs and use cases.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- What is a persistent object cache and how can one implement it?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
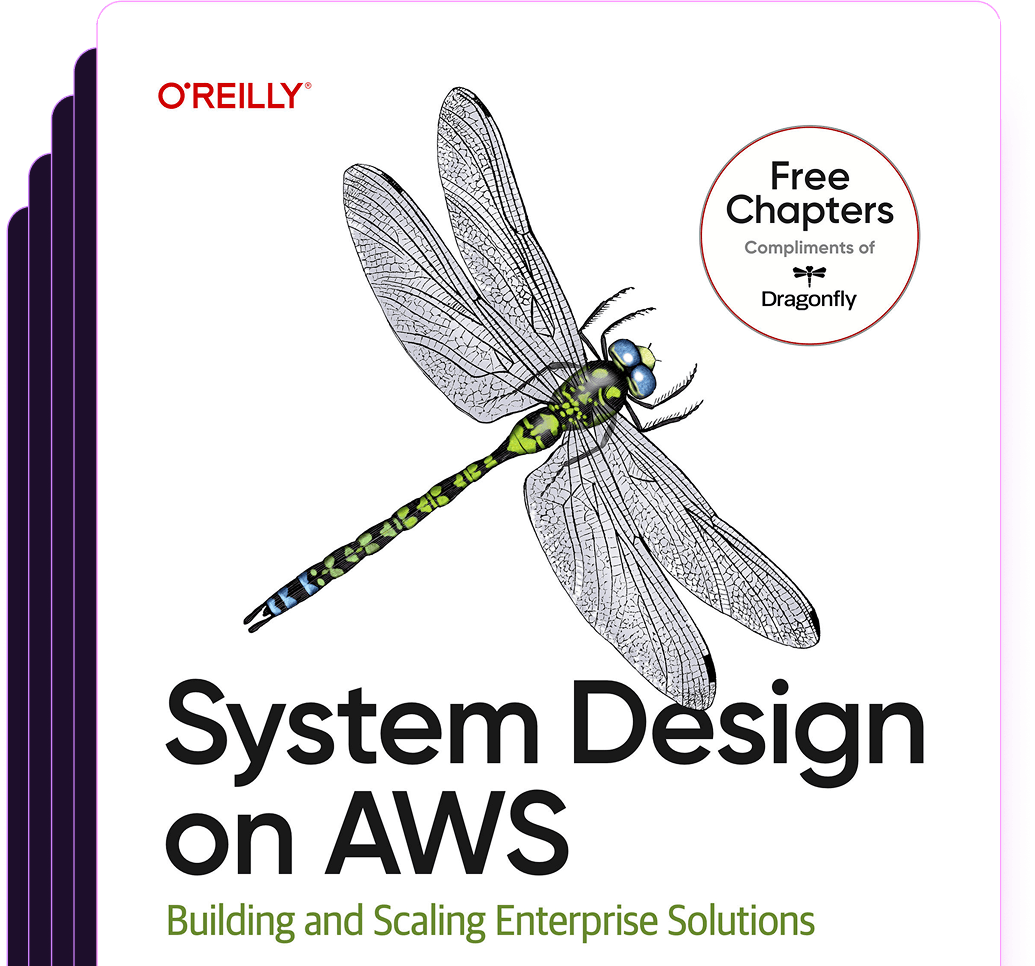
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost