Question: How to view ElastiCache data?
Answer
To view ElastiCache data, you can use the command-line interface or a client library. Here are some steps to view data using the command-line interface:
- Install the ElastiCache command-line interface (CLI) if you haven't already done so. You can find instructions on how to do this in the ElastiCache documentation.
- Connect to your cluster using the CLI by running the following command and replacing the placeholders with your values:
```
aws elasticache describe-cache-clusters --cache-cluster-id <your-cluster-id> --show-cache-node-info | grep "^ADDRESS" | awk '{print $2}'
```
- Once you have connected to your cluster, you can use the
redis-cli
tool to view data. For example, to view all keys in your Redis cache, run the following command:
```
redis-cli -h <your-hostname> -p <your-port> KEYS *
```
This will return a list of all keys currently stored in your cache.
- To view a specific key, run the following command, replacing
<key>
with the name of the key you want to view:
```
redis-cli -h <your-hostname> -p <your-port> GET <key>
```
This will return the value associated with the specified key.
If you prefer to use a client library instead of the command-line interface, you can use one of the many available libraries for your preferred programming language. For example, if you are using Python, you can use the redis
library to connect to your ElastiCache cluster and view data. Here's an example:
import redis
# Connect to the Redis cache
r = redis.Redis(
host='<your-hostname>',
port=<your-port>,
password='<your-password>'
)
# View all keys in the cache
keys = r.keys('*')
for key in keys:
print(key)
# View a specific key
value = r.get('<key>')
print(value)
This will connect to your ElastiCache cluster using the redis
library, and then allow you to view all keys or a specific key in the cache.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common ElastiCache Questions (and Answers)
- How to configure ElastiCache in AWS?
- Is ElastiCache stateless?
- What is ElastiCache Replication Group?
- When to use ElastiCache?
- Can ElastiCache store session data?
- How to improve ElastiCache performance?
- Can't connect to ElastiCache Redis
- Is ElastiCache a database?
- How to clear Elasticache?
- How many ElastiCache nodes do I need?
- Does ElastiCache work with RDS?
- Can ElastiCache be used with DynamoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
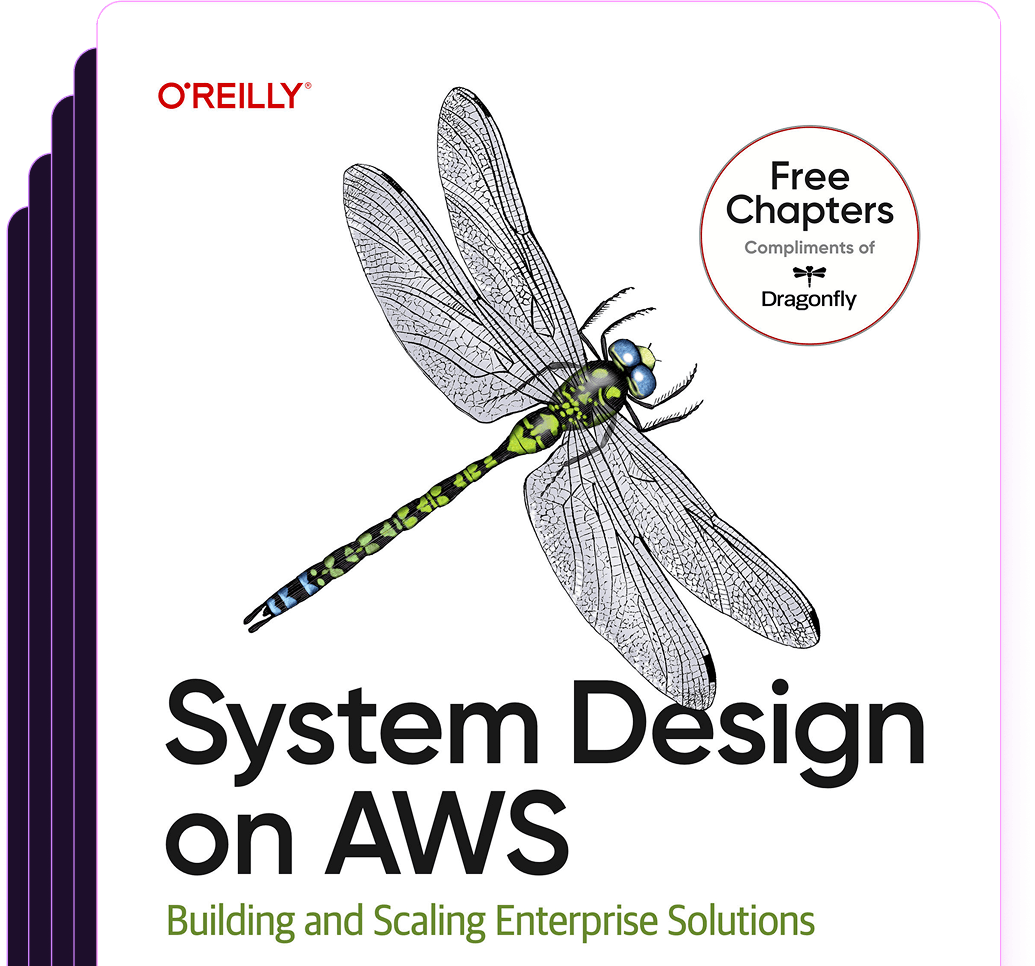
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost