Node Redis Bulk Get (Detailed Guide w/ Code Examples)
Use Case(s)
In Node.js applications, we often need to get multiple keys from a Redis store at once. This can be accomplished using the mget
command, which stands for 'Multi Get'. It allows you to retrieve the values of all specified keys.
Code Examples
- Basic Usage:
const redis = require('redis');
const client = redis.createClient();
client.mget(['key1', 'key2', 'key3'], function(err, reply) {
console.log(reply); // will print the values of key1, key2 and key3
});
This example shows how you can use mget
to fetch the values of multiple keys ('key1', 'key2', 'key3') at once.
- With Promise:
const { promisify } = require('util');
const redis = require('redis');
const client = redis.createClient();
const getAsync = promisify(client.mget).bind(client);
getAsync(['key1', 'key2', 'key3']).then(console.log).catch(console.error);
In this example, we're wrapping the mget
call with Node's built-in promisify
utility, allowing us to work with Promises instead of callbacks.
Best Practices
- Always handle potential errors in your callback or promise chain to prevent any unhandled exceptions.
- Be mindful about the size of the data you are requesting, as getting too many keys at once could potentially slow down your application.
Common Mistakes
- Not checking if the keys exist before trying to get them. This will return
null
values for those keys, which may not be the expected behavior in your application.
FAQs
- Can I use wildcard characters with mget? No. The Redis
mget
command does not support wildcards. If you need to get all keys matching a pattern, you need to first use thekeys
command to get the keys and then usemget
. - What happens if some of the keys do not exist? If some of the keys don't exist, Redis will return
null
for those keys.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
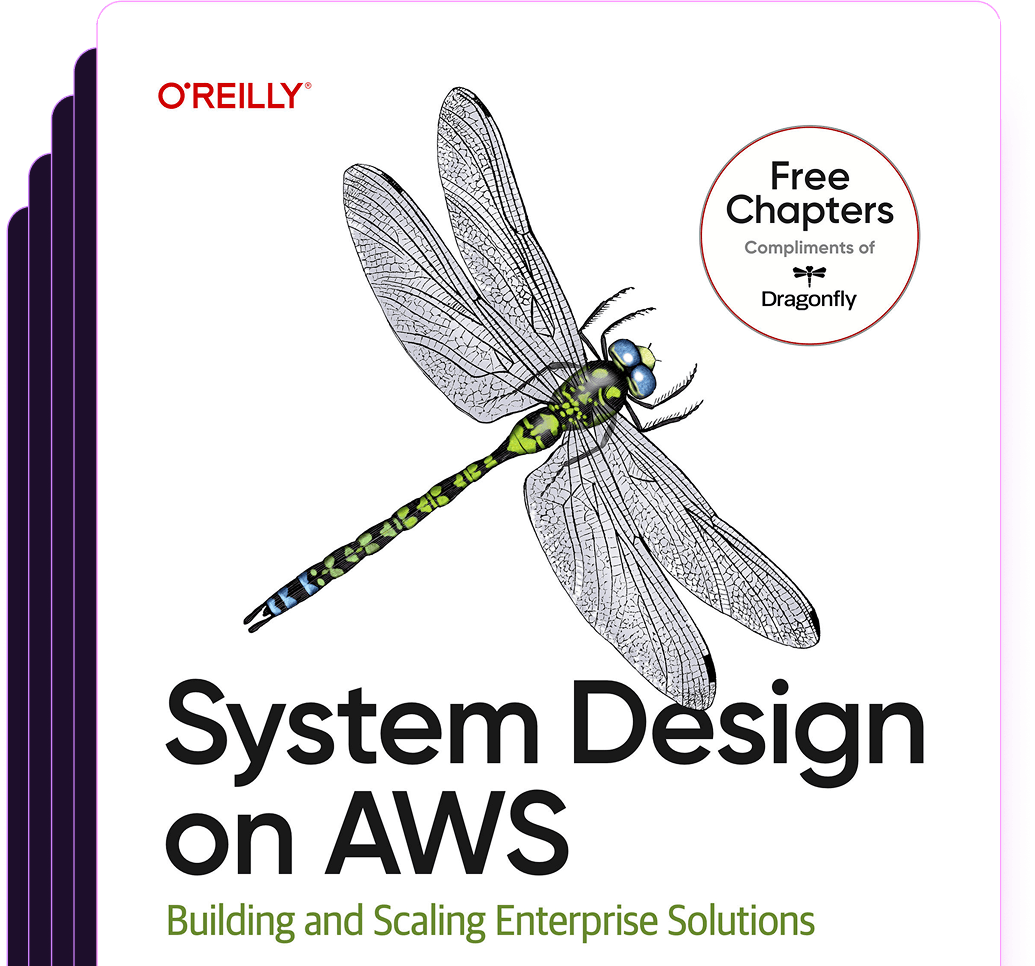
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost