Node Redis: Get All Keys Starting With (Detailed Guide w/ Code Examples)
Use Case(s)
Getting all keys starting with a certain prefix can be useful when you want to operate on a subset of data stored in Redis. For instance, if your application uses prefixes to categorize data (e.g., "user:" for user-related data, "session:" for session data), you might need to fetch all keys related to a particular category.
Code Examples
Example 1: The following script uses the KEYS
command to get all keys starting with 'user:' in a Redis database. CODE_BLOCK_PLACEHOLDER_0
In this example, the pattern 'user:*' is used with the keys
command to find all keys that start with 'user:'. The matching keys are then logged to the console.
Best Practices
While the KEYS
command can be very useful, it should be used with caution in a production environment as it may adversely affect performance due to its O(N) time complexity. A better approach would be to maintain an index of keys or use the SCAN
command which provides a cursor-based iterator that divides the work among multiple calls.
Common Mistakes
A common mistake is not considering the impact of the KEYS
command on performance, particularly for large data sets. It's a blocking operation that can potentially stall your Redis server if you have many keys.
FAQs
Q: Can I use the KEYS
command in a production environment? A: While it's technically possible, it's not recommended due to the potential performance impact. Consider using SCAN
instead.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get Hash Values at Key
- Node Redis: Get Current Memory Usage
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
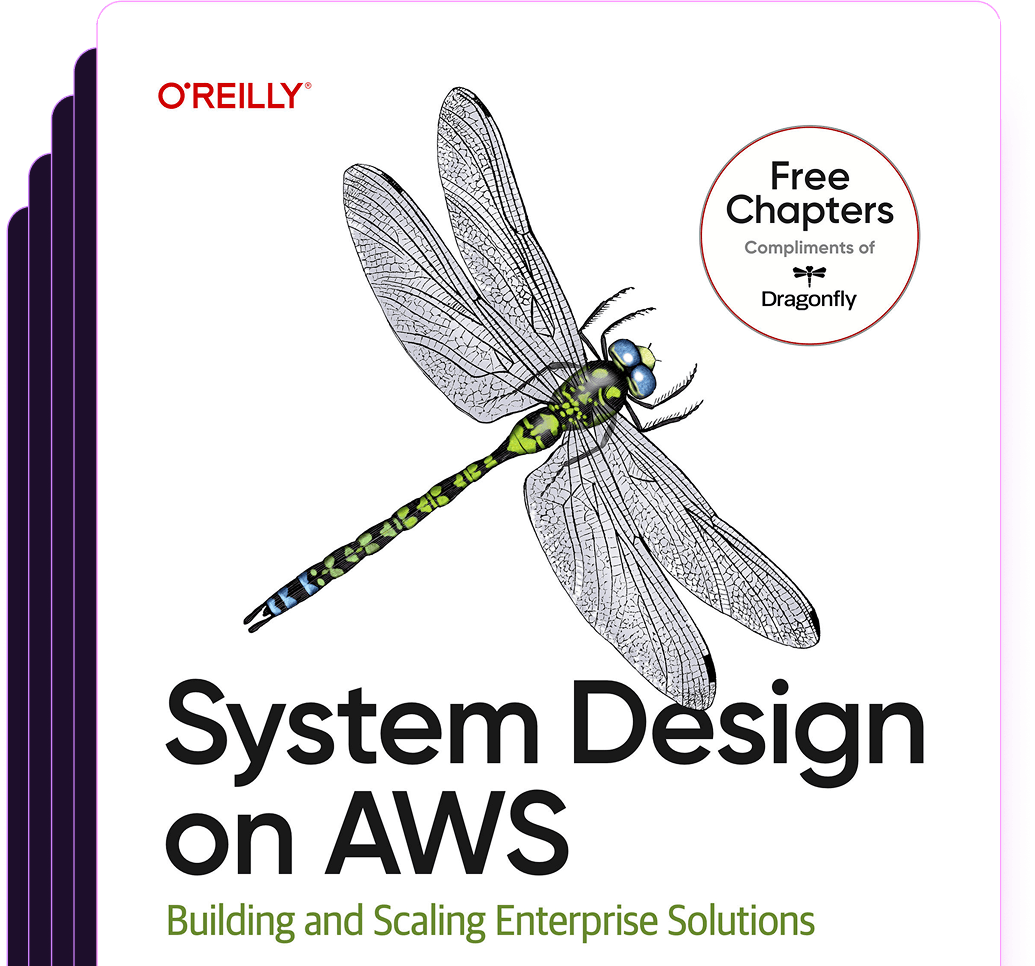
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost