Handling 'WRONGTYPE Operation' Error in Node Redis (Detailed Guide w/ Code Examples)
Use Case(s)
'WRONGTYPE Operation against a key holding the wrong kind of value' is an error that occurs when you try to perform an operation on a Redis key which has a different data type than expected. In Node.js, using redis.get
to retrieve a list, set, or hash will lead to this issue.
Code Examples
Here's a simple example illustrating the WRONGTYPE error:
const redis = require('redis');
const client = redis.createClient();
client.lpush('myList', 'item1', function(err, reply) {
console.log(reply);
});
client.get('myList', function(err, reply) {
if (err) {
console.log(err);
} else {
console.log(reply);
}
});
In this scenario, we're pushing an item into a list, then trying to fetch it using the get
method, which is meant for strings. This will result in the WRONGTYPE error.
Correct usage should be as follows:
const redis = require('redis');
const client = redis.createClient();
client.lpush('myList', 'item1', function(err, reply) {
console.log(reply);
});
client.lrange('myList', 0, -1, function(err, reply) {
if (err) {
console.log(err);
} else {
console.log(reply);
}
});
Here, instead of get
, we use lrange
which is the correct operation for a list.
Best Practices
Always ensure that you're using the correct Redis command for the respective data type. Redis provides separate commands for string (get
, set
), hash (hget
, hset
), list (lpush
, lrange
), etc. Using a wrong command can lead to the WRONGTYPE error.
Common Mistakes
- Misunderstanding Redis data types and corresponding commands: This can lead to the WRONGTYPE error. If you've created a key as a Hash, List, Set, or Sorted Set, you cannot access it as if it were a String.
- Not handling errors in your Node.js code: The Node Redis client will return an error when you attempt a command against the wrong data type. It's important to handle these errors to prevent them from causing unexpected behavior in your application.
FAQs
- Can I change the data type of a key in Redis? No, once a key is assigned a certain data type, it stays that way until it's deleted. If you need to change the type, you have to delete the key and recreate it with the desired type.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Node Redis: Get Replica
- Node Redis Get Key
- Node Redis: Get All Keys
- Node Redis: Get First 10 Keys
- Node Redis: Get All Keys and Values
- Node Redis: Get Length of List
- Get All Hash Keys with Redis in Node.js
- Node Redis: Get All Keys Matching Pattern
- Node Redis: Get Keys by TTL
- Node Redis: Getting All Databases
- Getting Master Status in Node Redis
- Node Redis: Get Number of Subscribers
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
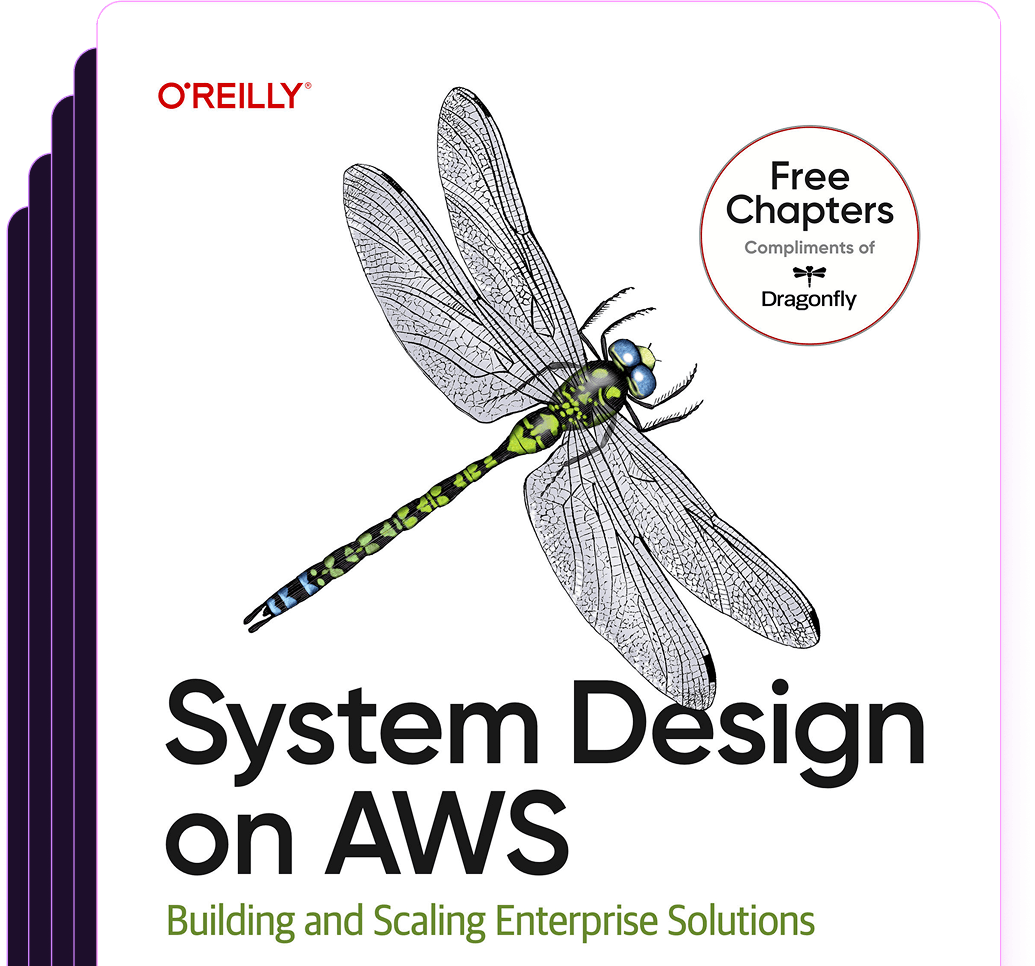
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost