Redis XTRIM in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The XTRIM
command in Redis is commonly used in the context of stream data structure. It's primarily employed to limit the length of a stream to the last N items. This can be useful in situations where you want to control the amount of memory your streams are consuming, typically in a scenario where you only care about recent data.
Code Examples
In this section, we'll review how to use the XTRIM
command using the Python Redis library (redis-py
).
Let's assume that we have a stream named "mystream". Here's an example of how to trim it:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Add some items to the stream
for i in range(100):
r.xadd("mystream", {"key": f"value{i}"})
# Trim the stream to the last 50 items
r.xtrim("mystream", 50)
In this example, the xtrim
command is being used to limit the size of the stream "mystream" to the last 50 items. After executing this code, "mystream" will only retain the most recent 50 entries.
Best Practices
It's recommended to use the XTRIM
command judiciously because it irreversibly removes data from the stream. Before using XTRIM
, make sure that old data is no longer needed or that it has been properly archived if necessary.
When dealing with highly important data, consider storing backups before performing operations that modify or delete data.
Common Mistakes
A common mistake is to forget that the XTRIM
command trims items from the start of the stream. It's important to bear in mind that you won't be able to recover any data removed by the XTRIM
command.
Another mistake is not handling exceptions when interacting with Redis - always include exception handling logic around your Redis interactions to manage potential connection issues or command errors.
FAQs
1. Is it possible to undo an XTRIM
operation?
No, once the XTRIM
operation has been performed, the data it removed is permanently deleted.
2. Can I use XTRIM
on other Redis data structures like lists or sets?
No, XTRIM
is specifically designed for stream data structure.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
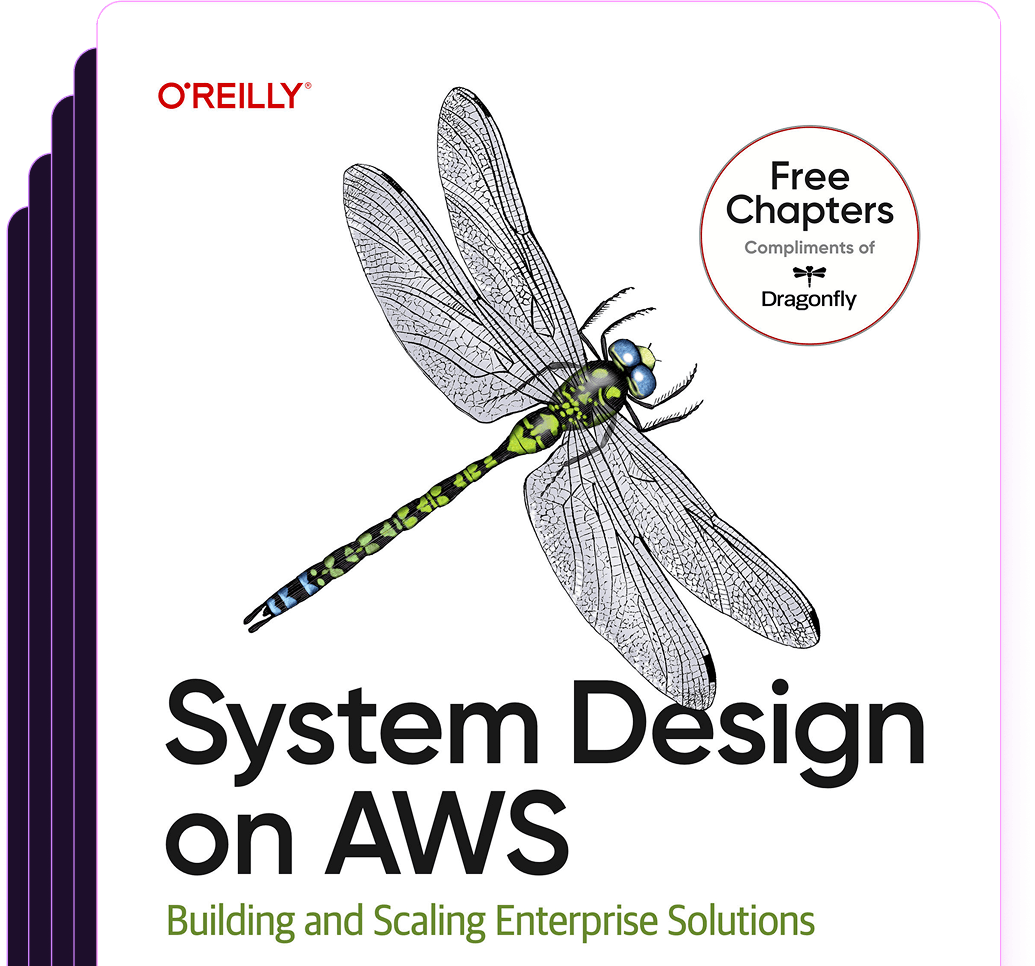
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost